Whenever you publish a new version of an existing workflow, only processes created after that will use the new definition. The existing in-flight process instances will keep using the old workflow definition and not the new one. This is the default behavior in APS, and you could find yourself with multiple instances running different process definition. However, it’s possible to update old instances to use the latest process definition.
You can achieve this using the REST API and making multiple calls (please refer to APS API Explorer if you want to pursue this approach)
You can also achieve this programmatically directly from a script task using the activiti-app following the next steps.
- Create a new process and include just a script task on it
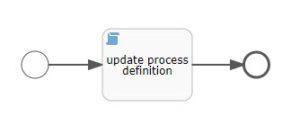
- Set the script task language as groovy
- Add the following code to the script task
import org.activiti.engine.impl.cmd.SetProcessDefinitionVersionCmd; import org.activiti.engine.repository.ProcessDefinition; import org.activiti.engine.runtime.ProcessInstance; import org.activiti.engine.impl.interceptor.CommandConfig; String YOUR_DEFINITION_NAME = "your_definition_name"; println("Updating Process Instances Definition..."); // Get the latest version ProcessDefinition processDefinition = execution.getEngineServices().getRepositoryService().createProcessDefinitionQuery().latestVersion().processDefinitionKey(YOUR_DEFINITION_NAME).singleResult(); if(processDefinition == null) { // If processDefinition is null, check if YOUR_DEFINITION_NAME matches any of the definition listed from the system List<ProcessDefinition> processDefinitionList = execution.getEngineServices().getRepositoryService().createProcessDefinitionQuery().active().latestVersion().list(); println("Process definition key NOT FOUND"); println("Total Process definition found: " + processDefinitionList.size()); // List all the process definitions name for(ProcessDefinition oneDefinition : processDefinitionList) { println("Definition key: " + oneDefinition.getKey()); } } else { println("Latest Process Definition Version: " + processDefinition.getVersion()); long counter = execution.getEngineServices().getRuntimeService().createProcessInstanceQuery().active().processDefinitionKey(YOUR_DEFINITION_NAME).count(); List<ProcessInstance> processInstanceList = execution.getEngineServices().getRuntimeService().createProcessInstanceQuery().active().processDefinitionKey(YOUR_DEFINITION_NAME).list(); // double check, both numbers should match println("Total process instances: " + counter); println("Total process instances retrieved: " + processInstanceList.size()); int index = 1; for (ProcessInstance oneInstance : processInstanceList) { try { CommandConfig commandConfig = new CommandConfig(); // Execute the command asynchronously to prevent that one error updating a process instance stops the entire batch execution.getEngineServices().getProcessEngineConfiguration().getAsyncExecutor().getCommandExecutor().execute(commandConfig.transactionNotSupported(), new SetProcessDefinitionVersionCmd(oneInstance.getId(), processDefinition.getVersion())); println(index++ + " - Process instance UPDATED: " + oneInstance.getId() + ", previous definition ID: " + oneInstance.getProcessDefinitionVersion()); } catch (Exception e) { println(index++ + " - Process instance FAILED: " + oneInstance.getId() + ", previous definition ID: " + oneInstance.getProcessDefinitionVersion() + ", Cause: " + e.getMessage()); } } println("Completed"); }
- Set the name (key) of your process definition into the variable YOUR_DEFINITION_NAME
- Create an app with the new process model and publish it
- Execute the app (start a process) and check the console output
Important Note: applying major changes in your process definition could make impossible to update from an old version to the new one. Activities ID must remain the same, from version to version, to let APS update the definition properly.